Design Chat App
Design and Implement a Chat App
.
Chat App
is an application that allows users to communicate with each other in real-time.
Examples: Slack, Discord, Telegram, WhatsApp.
Requirements
Functional Requirements
- Allow users to send and receive messages in real-time
- Support chat rooms with ability to manage, join and leave rooms
- Support attachments like images, videos, files, locations
- Support for voice and video calls
- User can share the message with other users
Non-Functional Requirements
- Low latency for sending and receiving messages (40-60ms)
- High availability (99.99% uptime)
- Scalability to handle a large number of users (10k+)
- Responsive design for various screen sizes (mobile, tablet, desktop)
- Support for internationalization (multiple languages)
- Ensure accessibility (screen readers, keyboard navigation)
- Minimize battery consumption, memory usage, and CPU resource consumption
High-Level Design / Architecture (Big Picture)
Let's draw a high level diagram including the Server part. It's important to to see the big picture and have an understading how these building blocks work with each other even if you are a Frontend Developer.
The High Level Design of Chat App should include the following blocks:
Core Architectural Components
- Clients: Applications for web, mobile, and desktop platforms.
- Communication Layer:
- WebSocket for persistent real-time connections.
- REST API Gateway for standard HTTP interactions.
- Services:
- Auth Service: Handles user authentication and authorization.
- Chat Service: Manages chat rooms and user interactions.
- Message Service: Processes, stores, and retrieves messages.
- Notification Service: Sends push notifications to clients.
- Data Storage:
- User, Chat, and Media databases.
- Cache layers for improved performance.
- Message queues for asynchronous processing (e.g., Kafka).
Technologies:
- Database: Use Cassandra for scalability and Redis for caching.
- Media Storage: Blob storage for attachments, backed by a CDN.
- Queue Systems: Kafka for handling events and message processing.
- Load Balancers: Ensure equal traffic distribution.
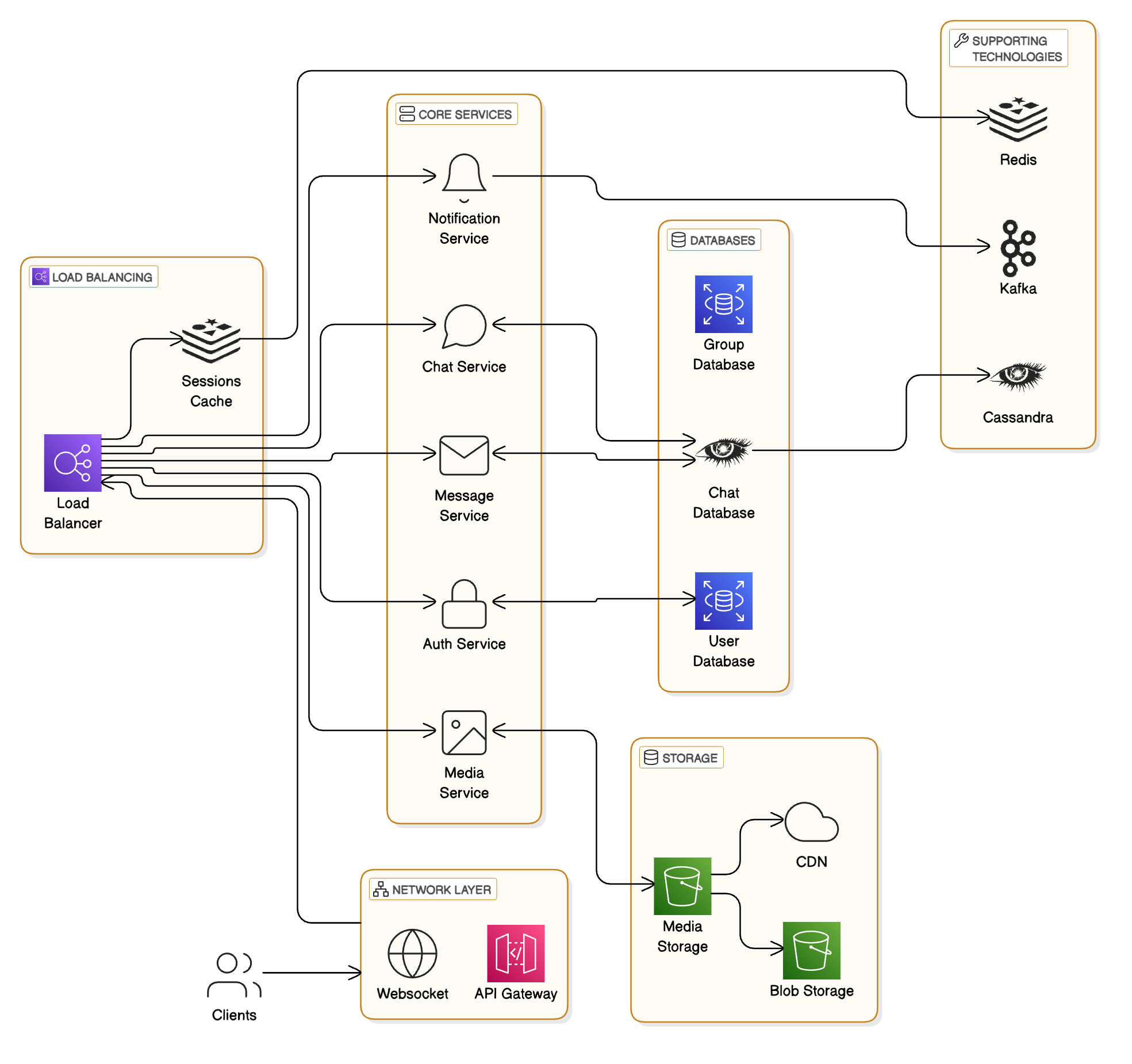
Frontend Components
The frontend application should be organized into the following components:
- Chat Window: Displays messages and provides a text input box.
- Chat Room List: Lists available chat rooms for navigation.
- User List: Shows users currently in a selected chat room.
- Search Bar: Allows searching for chat rooms or users.
- Sidebar: Provides navigation and quick actions.
Here is a component's structure in the codebase:
src/
├── components/
│ ├── ContactList.js
│ ├── ChatRoomList.js
│ ├── ChatWindow/
│ │ ├── MessageList.js
│ │ ├── TypingIndicator.js
│ │ └── InputBox.js
│ ├── Sidebar/
│ │ ├── ChatList.js
│ │ └── SearchBar.js
│ └── Settings/
├── context/
├── hooks/
├── services/
│ ├── api.js
│ ├── websocket.js
│ └── auth.js
├── styles/
└── utils/
API Endpoints
The chat app system should expose the following API endpoints:
GET /chat-rooms
GET /chat-rooms/:id
POST /chat-rooms
PUT /chat-rooms/:id
DELETE /chat-rooms/:id
GET /users
GET /users/:id
POST /users
PUT /users/:id
DELETE /users/:id
GET /messages
GET /messages/:id
POST /messages
PUT /messages/:id
DELETE /messages/:id
GET /online-users
GET /offline-users
GET /search
API Data Models
The chat app system should use the following data models:
interface User {
id: string;
name: string;
email: string;
phone: string;
avatarURL: string;
}
// there are two options: message to user and massage to chat room
interface Message {
id: string;
userId: string;
chatRoomId: string;
text: string;
createdAt: Date;
attachments?: Attachment[];
}
interface Attachment {
id: string;
message_id: string;
type: 'link' | 'video' | 'audio' | 'image' | 'file';
url: string;
}
interface ChatRoom {
id: string;
name: string;
users: User[];
messages: Message[];
}
Security
The chat app system should implement the following security measures:
- Users should be
authenticated
to access the chat app. - Users should be
authorized
to access the chat app. - Messages should be
encrypted
to ensure privacy. - Personal information should be
protected
from unauthorized access. Only authorized
users should be able to access the chat app.Limit the number of requests
per user to prevent abuse and DDoS attacks.- Logging: Log user actions to track user behavior and identify security issues.
Accessibility
The chat app system should be designed with accessibility in mind:
- Use semantic HTML elements for screen readers.
- Provide keyboard navigation for users with motor disabilities.
- Ensure color contrast for users with visual impairments.
- Use ARIA attributes for interactive elements.
- Provide text alternatives for non-text content.
- Test the app with accessibility tools and real users.
Summary
The Chat App should be a secure, scalable, and user-friendly application enabling real-time communication. By adhering to functional and non-functional requirements, designing modular frontend components, and ensuring robust backend support, the app can deliver a seamless user experience.