Events: bubbling, capturing, propagation, delegation
This post explores the concept of event propagation in JavaScript, covering the three main phases: event capturing, event target, and event bubbling. We delve into how events travel through the DOM, the specifics of handling events during each phase, and the technique of event delegation for managing events efficiently on parent containers. This is essential reading for understanding how JavaScript interacts with web pages, making it crucial for both budding and seasoned developers.
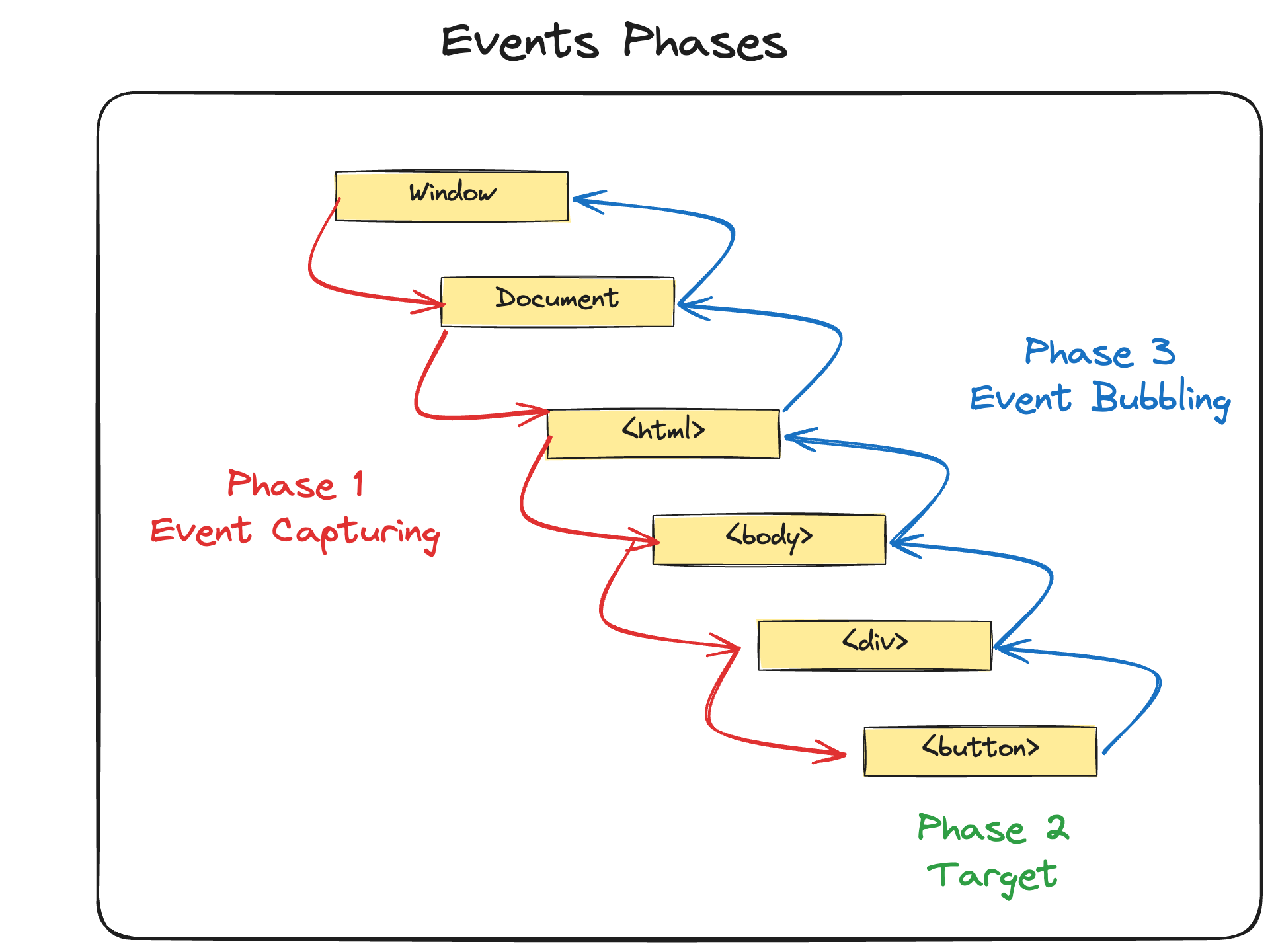
Event Propagation
Event propagation in JavaScript is the mechanism that determines how events travel through the Document Object Model (DOM) tree when an interaction happens on a web page. It dictates the order in which event listeners attached to different elements in the DOM hierarchy are triggered.
There are three different phases during the lifecycle of a Event Propagation: Event Capturing, Event Target, and Event Bubbling. Let's explore each one.
Event Capturing
This is the initial phase where the event travels from the window
object down to the target element. During this phase, event handlers set to handle events in the capturing phase will trigger.
To enable event capturing
in JavaScript, you need to set an event listener and specify that you want it to execute during the capturing phase. You can do this by setting the third argument of addEventListener
to true
el.addEventListener('click', (evt) => {
console.log('Click event captured on ' + evt.currentTarget.tagName);
}, true);
Event Target
The second phase, called the target phase, begins immediately after the capturing phase ends. It marks the end of capturing and the beginning of bubbling.
Any event handlers attached directly to the target are executed when the event reaches the target element. This phase applies to both the capturing and bubbling phases.
Event Bubbling
The bubbling phase
is the reverse of the capturing phase
, and events bubble up from the target element to the global window object. By default, all events added with addEventListener
are in the bubble phase
.
During this phase, event handlers set to handle events in the bubbling phase will trigger.
How to stop Event Bubbling
button.addEventListener('click', (event) => {
event.stopPropagation()
console.log("button was clicked")
})
Event Delegation
Event delegation in JavaScript is a technique where you use a single event listener to manage all events of a specific type that might occur on various child elements within a parent container. This approach is efficient because it reduces the number of event listeners you need to attach to elements, and it can handle events on elements that are dynamically added to the page.
How Event Delegation works
Benefits
- You only need one event listener rather than one for each child,
reducing the memory footprint
. - You can add new elements to the DOM, and they will
automatically be handled by the existing event listener
without needing to attach new listeners. Managing one event listener
is often simpler than managing many separate listeners spread across various elements.
Example
<ul id="itemList">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<!-- More items can be added dynamically -->
</ul>
// Get the parent <ul> element
const list = document.getElementById('itemList');
// Attach the event listener to the parent
list.addEventListener('click', function(event) {
// Check if the clicked element is a <li>
if (event.target.tagName === 'li') {
console.log('You clicked on:', event.target.textContent);
}
});
Summary
Event propagation
is the mechanism that determines how events travel through the DOM tree. It consists of three phases: event capturing, event target and event bubbling.Event capturing
is the initial phase where the event travels from thewindow
object down to the target element.Event target
begins immediately after the capturing phase ends. It marks the end of capturing and the beginning of bubblingEvent bubbling
is the reverse of Event capturing phase. In this phase events bubble up from the target element to the global window object.Event delegation
is a pattern where we delegate the responsibility of handling an event to a parent element.