Design News Feed
Design and Implement a News Feed
.
News Feed
is a feature that displays a list of news articles to users.
It allows users to scroll through the list, view more details about an article, search for articles by keyword, share articles on social media, like or comment on articles, and sort articles by date, popularity, or relevance.
News Feed
is a common feature in social media platforms and news websites like: Instagram, Facebook and Reddit.
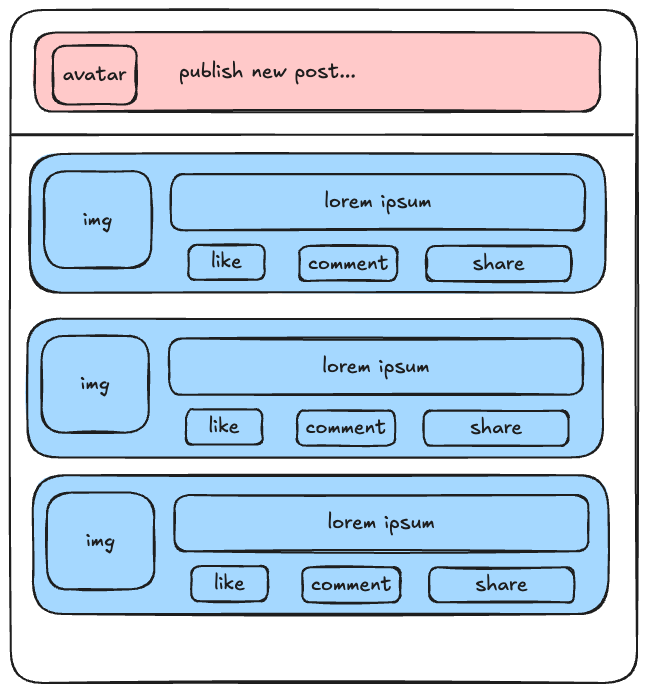
Requirements
Functional Requirements
- Display a list of news
- Allow users to scroll through the list
- Show a loading indicator while fetching articles
- Allow users to click on an article to view more details
- Provide a search bar to filter articles by keyword
- Allow users to share articles on social media
- Support pagination for large datasets
- Display images and text for each article
- Allow users to like or comment on articles
- Show the number of likes and comments for each article
- Allow users to sort articles by date, popularity, or relevance
Non Functional Requirements
- Low latency for fetching articles
- High availability
- Scalable to handle large number of users
- Responsive design for different screen sizes
- Support for internationalization
- Support for accessibility (screen readers, keyboard navigation)
- Support for mobile devices
High Level Design
The news feed system can be divided into the following components:
- Frontend: The user interface that displays news articles and handles user interactions.
- Backend: The server that fetches news articles from a database and serves them to the frontend.
- Database: The storage system for news articles and user data.
- Cache: An in-memory cache to store frequently accessed articles and reduce latency.
- API Gateway: A service that routes requests to the appropriate backend service.
- Load Balancer: Distributes incoming traffic across multiple backend servers.
- CDN: Delivers static assets like images, CSS, and JavaScript files to users.
- Monitoring: Tracks system performance, logs, and metrics to identify issues and optimize performance.
Frontend Components
The frontend application should be organized into the following components:
News Feed
: Displays a list of news articles with images and text.Article Details
: Shows more information about a selected article.- Search Bar: Filters articles based on user input.
- Loading Indicator: Indicates that articles are being fetched.
- Pagination: Allows users to navigate through multiple pages of articles.
- Share Buttons: Enable users to share articles on social media platforms.
- Like Button: Allows users to like an article.
- Comment Section: Displays comments and allows users to add new comments.
- Sorting Options: Lets users sort articles by date, popularity, or relevance.
API Endpoints
The news feed system should expose the following API endpoints:
GET /posts
GET /posts/:id
POST /posts/:id/like
POST /posts/:id/comment
GET /posts/search?q=:query
GET /posts/sort?by=:field
Data Models Schema
The database should store the following entities:
- Posts
interface Post {
id: string;
title: string;
content: string;
img_url: string;
created_at_: Date;
likes: number;
comments: Comment[];
author: User;
}
- Users
interface User {
user_id: number;
username: string;
email: string;
profile_pic: string;
created_at: Date;
updated_at: Date;
posts: Post[];
comments: Comment[];
likes: Like[];
}
- Comments
interface Comment {
comment: string;
user: User;
created_at: Date;
}
- Likes
interface Like {
user: User;
created_at: Date;
}
Performance
To improve performance, the news feed system can implement the following optimizations:
- Caching: store frequently accessed articles in a cache to reduce latency.
- Lazy Loading: load images and content as the user scrolls to improve initial page load time.
- Pagination: limit the number of articles fetched per request to reduce server load.
- Throttling: limit the number of requests per user to prevent abuse and improve system stability.
- Debouncing: delay search requests until the user has finished typing to reduce unnecessary API calls.
Accessibility
To ensure accessibility, the news feed system should adhere to the following guidelines:
- Keyboard Navigation: allow users to navigate the news feed using keyboard shortcuts.
- Screen Reader Support: provide alt text for images and ARIA attributes for interactive elements.
- Color Contrast: ensure text and background colors have sufficient contrast for readability.
- Focus Indicators: highlight focused elements for users who navigate using a keyboard.
- Semantic HTML: use semantic HTML elements to improve screen reader compatibility.
Security
To protect user data and prevent unauthorized access, the news feed system should implement the following security measures:
- Authentication: Require users to log in to access their account and post comments or likes.
- Authorization: Restrict access to sensitive endpoints like posting comments or likes to authenticated users.
- Input Validation: Sanitize user input to prevent SQL injection, XSS attacks, and other security vulnerabilities.
- HTTPS: Encrypt data transmitted between the client and server to prevent eavesdropping.
- Rate Limiting: Limit the number of requests per user to prevent abuse and DDoS attacks.
Summary
Designing a news feed system involves creating a user-friendly interface, optimizing performance, ensuring accessibility, and implementing security measures to protect user data. By following best practices and guidelines, you can build a scalable and reliable news feed system that meets the needs of your users.