Implement Tic Tac Toe Game
Design and implement Tic Tac Toe Game.
Tic Tac Toe
is a two-player game where each player takes turns to mark a cell in a 3x3 grid. The player who succeeds in placing three of their marks in a horizontal, vertical, or diagonal row wins the game.
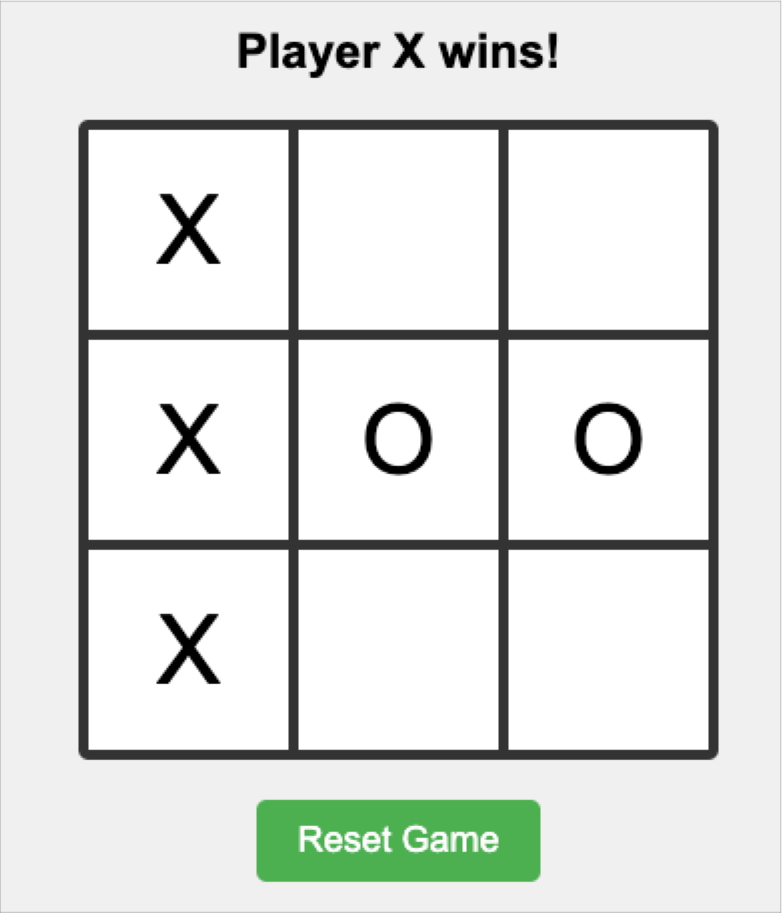
Solution
HTML
Let's start with defining the layout of the game.
We will need 3 elements:
status element
to display the current player's turnboard element
to display the 3x3 gridreset button
to restart the game
<body>
<div class="status" id="status">Player X's turn</div>
<div class="board" id="board"></div>
<button class="reset-button" id="resetButton">Reset Game</button>
</body>
Javascript
Now, let's implement the game logic using JavaScript.
We will create a TicTacToe
class that will handle the game state, player turns, and check for a winner.
- Let's create a board that represents the 3x3 grid. We will use an array of 9 elements to store the current state of the board
- We will keep track of the current player (X or O) and update it after each move
- We will add event listeners to the board cells to handle user input.
- We will check for a winner after each move and display the result.
- We will add a reset button to restart the game.
class TicTacToe {
constructor() {
this.board = Array(9).fill(null);
this.currentPlayer = "X";
this.gameEnded = false;
this.boardElement = document.getElementById("board");
this.statusElement = document.getElementById("status");
this.resetButton = document.getElementById("resetButton");
this.initializeBoard();
this.addEventListeners();
}
initializeBoard() {
this.boardElement.innerHTML = "";
for (let i = 0; i < 9; i++) {
const cell = document.createElement("button");
cell.classList.add("cell");
cell.dataset.index = i;
this.boardElement.appendChild(cell);
}
}
addEventListeners() {
this.boardElement.addEventListener("click", (e) => {
if (e.target.classList.contains("cell")) {
this.makeMove(e.target.dataset.index);
}
});
this.resetButton.addEventListener("click", () => this.resetGame());
}
makeMove(index) {
if (this.board[index] || this.gameEnded) return;
this.board[index] = this.currentPlayer;
const cell = this.boardElement.children[index];
cell.textContent = this.currentPlayer;
if (this.checkWinner()) {
this.gameEnded = true;
this.statusElement.textContent = `Player ${this.currentPlayer} wins!`;
return;
}
if (this.board.every((cell) => cell !== null)) {
this.gameEnded = true;
this.statusElement.textContent = "It's a draw!";
return;
}
this.currentPlayer = this.currentPlayer === "X" ? "O" : "X";
this.statusElement.textContent = `Player ${this.currentPlayer}'s turn`;
}
checkWinner() {
const winPatterns = [
[0, 1, 2], [3, 4, 5], [6, 7, 8], // Rows
[0, 3, 6], [1, 4, 7], [2, 5, 8], // Columns
[0, 4, 8], [2, 4, 6], // Diagonals
];
return winPatterns.some((pattern) => {
const [a, b, c] = pattern;
return this.board[a] && this.board[a] === this.board[b] && this.board[a] === this.board[c];
});
}
resetGame() {
this.board = Array(9).fill(null);
this.currentPlayer = "X";
this.gameEnded = false;
this.statusElement.textContent = "Player X's turn";
Array.from(this.boardElement.children).forEach((cell) => {
cell.textContent = "";
});
}
}
// Initialize the game
new TicTacToe();
Styles
Add some basic styles to make the game look better.
body {
display: flex;
flex-direction: column;
align-items: center;
background-color: #f0f0f0;
}
.status {
margin: 20px 0;
font-size: 24px;
font-weight: bold;
}
.board {
display: grid;
grid-template-columns: repeat(3, 100px);
gap: 5px;
background-color: #333;
padding: 5px;
border-radius: 5px;
}
.cell {
width: 100px;
height: 100px;
background-color: white;
border: none;
font-size: 48px;
cursor: pointer;
display: flex;
align-items: center;
justify-content: center;
transition: background-color 0.2s;
}
.cell:hover {
background-color: #f0f0f0;
}
.cell:disabled {
cursor: not-allowed;
}
.reset-button {
margin-top: 20px;
padding: 10px 20px;
font-size: 18px;
background-color: #4caf50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.2s;
}
.reset-button:hover {
background-color: #45a049;
}
Demo
You can see the live demo of the Tic Tac Toe game here.
Summary
In this article, we implemented a simple Tic Tac Toe game using HTML, CSS, and JavaScript.
The game allows two players to take turns marking cells in a 3x3 grid. The player who succeeds in placing three of their marks in a horizontal, vertical, or diagonal row wins the game.
We used event listeners to handle user input and check for a winner after each move. We also added a reset button to restart the game.